Salesforce are on a mission to make accessing off platform data and web services as easy as possible. This helps keep the user experience optimal and consistent for the user and also allows admins to continue to leverage the platforms tools such as Process Builder and Flow, even if the data or logic is not on the platform.
Starting with External Objects, they added the ability to see and also update data stored in external databases. Once setup, users can manipulate external records without leaving Salesforce, by staying within the familiar UI’s. With External Services, currently in Beta, they have extended this concept to external API services.
UPDATE: The ASCIIArt Service covered in this blog has since been updated to use the Swagger schema standard. However this blog is still a very useful introduction to External Services. Once you have read it, head on over to this blog!
In this blog lets first focus on the clicks-not-code steps you can repeat in your own org, to consume a live ASCII Art web service API i have exposed publicly. The API is simple, it takes a message and returns it in ASCII art format. The following steps result in a working UI to call the API and update a record.
After the clicks not code bit i will share how the API was built, whats required for compatibility with this feature and how insanely easy it is to develop Web Services in Heroku using Nodejs. So lets dive in to External Services!
Building an ASCII Art Converter in Lightning Experience and Flow
The above solution was built with the following configurations / components. All of which are accessible under the LEX Setup menu (required for External Services) and takes around 5 minutes maximum to get up and running.
- Named Credential for the URL of the Web Service
- External Service for the URL, referencing the Named Credential
- Visual Flow to present a UI, call the External Service and update a record
- Lightning Record Page customisation to embed the Flow in the UI
I created myself a Custom Object, called Message, but you can easily adapt the following to any object you want, you just need a Rich Text field to store the result in. The only other thing you need to know of course is the web service URL.
https://createasciiart.herokuapp.com
Can i use External Services with any Web Service then?
In order to build technologies that simplify what are normally things developers have to interpret and code manually. Web Service APIs must be documented in a way that External Services can understand. In this Beta release this is the Interagent schema standard (created by Heroku as it happens). Support for the more broadly adopted Swagger / OpenId will be added in the Winter release (Safe Harbour).
For my ASCII Art service above, i authored the Interagent schema based on a sample the Salesforce PM for this feature kindly shared, more on this later. When creating the External Service in moment we will provide a schema to this service.
https://createasciiart.herokuapp.com/schema
Creating a Named Credential
From the setup menu search for Named Credential and click New. This is a simple Web Service that requires no authentication. Basically provide only the part of the above URL that points to the Web Service endpoint.
Creating the External Service
Now for the magic! Under the Setup menu (only in Lightning Experience) search for Integrations and start the wizard. Its a pretty straight forward process, of selecting the above Named Credential, then telling it the URL for the schema. If thats not exposed by the service you want to use, you can paste a Schema in directly (which lets a developer define a schema yourself if one does not already exist).
Once you have created the External Service you can review the operations it has discovered. Salesforce uses the documentation embedded in the given schema to display a rather pleasing summary actually.
So what just happened? Well… internally the wizard wrote some Apex code on your behalf and implemented the Invocable Method annotations to enable that Apex code to appear in tools like Process Builder (not supported in Beta) and Flow. Pretty cool!
Whats more interesting for those wondering, is you cannot actually see this Apex code, its there but some how magically managed by the platform. Though i’ve not confirmed, i would assume it does not require code coverage.
Update: According to the PM, in Winter’18 it will be possible “see” the generated class from other Apex classes and thus reuse the generated code from Apex as well. Kind of like a Api Stub Generator.
Creating a UI to call the External Service via Flow
This simple Flow prompts the user for a message to convert, calls the External Service and updates a Rich Text field on the record with the response. You will see in the Flow sidebar the generated Apex class generated by the External Service appears.
The following screenshots show some of the key steps involved in setting up the Flow and its three steps, including making a Flow variable for the record Id. This is later used when embedding the Flow in Lightning Experience in the next step.

RecordId used by Flow Lightning Component

Assign the message service parameter
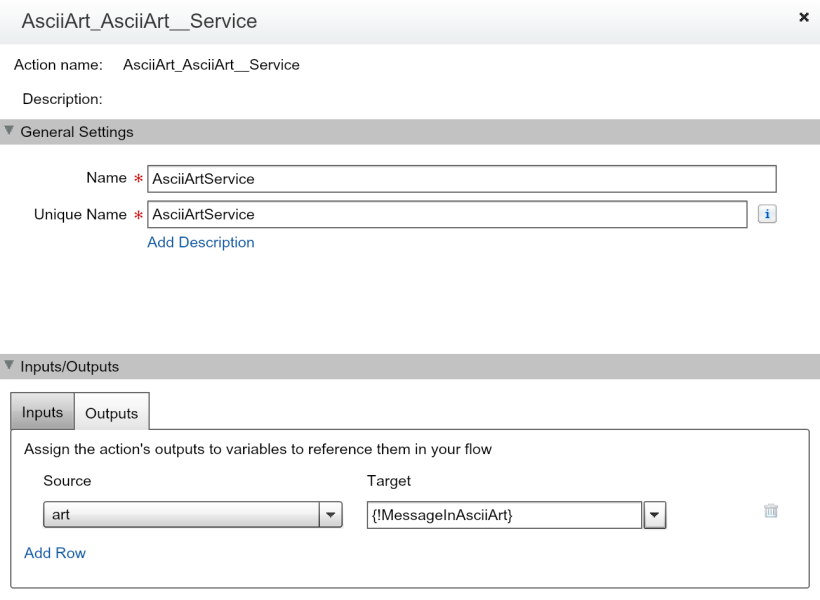
Assign the response to variable

Update the Rich Text field
TIP: When setting the ASCII Art service response into the field, i wrapped the value in the HTML elements, pre and code to ensure the use of a monospaced font when the Rich Text field displayed the value.
Embedding the Flow UI in Lightning Experience
Navigate to your desired objects record detail page and select Edit Page from the cog in the top right of the page to open the Lightning App Builder. Here you can drag the Flow component onto the page and configure it to call the above flow. Make sure to map the Flow variable for the record Id as shown in the screenshot, to ensure the current record is passed.
Thats it, your done! Enjoy your ASCII Art messages!
Creating your own API for use with External Services
Belinda, the PM for this feature was also kind enough to share the sample code for the example shown in TrailheaDX, from which the service in this blog is based. However i did wanted to build my own version to do something different from the credit example. Also extend my personal experience with Heroku and Nodejs more.
The NodeJS code for this solution is only 41 lines long. It runs up a web server (using the very easy to use hapi library), and registers a couple of handlers. One handler returns the statically defined schema.json file, the other implements the service itself. As side note, the joi library is an easy way add validation to the service parameters.
var Hapi = require('hapi'); var joi = require('joi'); var figlet = require('figlet'); // initialize http listener on a default port var server = new Hapi.Server(); server.connection({ port: process.env.PORT || 3000 }); // establish route for serving up schema.json server.route({ method: 'GET', path: '/schema', handler: function(request, reply) { reply(require('./schema')); } }); // establish route for the /asciiart resource, including some light validation server.route({ method: 'POST', path: '/asciiart', config: { validate: { payload: { message: joi.string().required() } } }, handler: function(request, reply) { // Call figlet to generate the ASCII Art and return it! const msg = request.payload.message; figlet(msg, function(err, data) { reply(data); }); } }); // start the server server.start(function() { console.log('Server started on ' + server.info.uri); });
I decided i wanted to explore the diversity of whats available in the Nodejs space, through npm. To keep things light i chose to have a bit of fun and quickly found an ASCIIArt library, called figlet. Though i soon discovered that npm had a library for pretty much every other use case i came up with!
Finally the hand written Interagent schema is also shown below and is reasonably short and easy to understand for this example. Its not all that well documented in layman’s terms as far as i can see. See my thoughts on this and upcoming Swagger support below.
{ "$schema": "http://interagent.github.io/interagent-hyper-schema", "title": "ASCII Art Service", "description": "External service example from AndyInTheCloud", "properties": { "asciiart": { "$ref": "#/definitions/asciiart" } }, "definitions": { "asciiart": { "title": "ASCII Art Service", "description": "Returns the ASCII Art for the given message.", "type": [ "object" ], "properties": { "message": { "$ref": "#/definitions/asciiart/definitions/message" }, "art": { "$ref": "#/definitions/asciiart/definitions/art" } }, "definitions": { "message": { "description": "The message.", "example": "Hello World", "type": [ "string" ] }, "art": { "description": "The ASCII Art.", "example": "", "type": [ "string" ] } }, "links": [ { "title": "AsciiArt", "description": "Converts the given message to ASCII Art.", "href": "/asciiart", "method": "POST", "schema": { "type": [ "object" ], "description": "Specifies input parameters to calculate payment term", "properties": { "message": { "$ref": "#/definitions/asciiart/definitions/message" } }, "required": [ "message" ] }, "targetSchema": { "$ref": "#/definitions/asciiart/definitions/art" } } ] } } }
Finally here is the package.json file that brings the whole node app together!
{ "name": "asciiartservice", "version": "1.0.0", "main": "server.js", "dependencies": { "figlet": "^1.2.0", "hapi": "~8.4.0", "joi": "^6.1.1" } }
Other Observations and Thoughts…
- Error Handling.
You can handle errors from the service in the usual way by using the Fault path from the element. The error shown is not all that pretty, but then in fairness there is not really much of a standard to follow here.
- Can a Web Service called this way talk back to Salesforce?
Flow provides various system variables, one of which is the Session Id. Thus you could pass this as an argument to your Web Service. Be careful though as the running user may not have Salesforce API access and this will be a UI session and thus will be short lived. Thus you may want to explore another means to obtain an renewable oAuth token for more advanced uses. - Web Service Callbacks.
Currently in the Beta the Flow is blocked until the Web Service returns, so its good practice to make your service short and sweet. Salesforce are planning async support as part of the roadmap however. - Complex Parameters.
Its unclear at this stage how complex a web service can be supported given Flows limitations around Invocable Methods which this feature depends on. - The future is bright with Swagger support!
I am really glad Salesforce are adding support for Swagger/OpenID, as i really struggled to find good examples and tutorials around Interagent. Really what is needed here is for the schema and code to be tied more closely together, like this!UPDATE: See my other blog entry covering Swagger support
Summary
Both External Objects and External Services reflect the reality of the continued need for integration tools and making this process simpler and thus cheaper. Separate services and data repositories are for now here to stay. I’m really pleased to see Salesforce doing what it does best, making complex things easier for the masses. Or as Einstein would say…“Everything should be made as simple as possible, but no simpler“.
Finally you can read more about External Objects here and here through Agustina’s and laterally Alba’s excellent blogs.
July 26, 2017 at 5:26 pm
Hi Andy,
Great article! I have a question. I have access to a few web services, but they have not provided any URL for the schema and I don’t have much control over that; would that still be possible to manually define the schema on our end or it is something that should be done by other party who’s providing the web services?
July 27, 2017 at 3:40 am
Yep it’s totally possible to do that. In the screenshot above in the wizard there is a checkbox to enable a field to paste in the schema rather than obtaining it via a URL. 👍🏻
July 27, 2017 at 7:18 am
Hi Andy,
It’s really descriptive article about the integration, but I want to ask is the same integration possible via Salesforce Classic as well.
July 27, 2017 at 7:19 am
The setup wizard is only in Lightning experience but the results and the runtime will be available and function in classic
August 11, 2017 at 3:17 pm
Thank you Sharing great article for API integration.
August 16, 2017 at 12:08 pm
Awesome article…quite detailed and i could do the POC at my end. Thanks Andy!
August 17, 2017 at 12:25 am
Excellent!
August 23, 2017 at 7:19 pm
Hi Andrew,
I have a question for you on DLRS. We are trying to do the following: We’re using Classy to manage our donations. We want to number sequentially the Classy Recurring Donor Opportunity records. This is a problem because the Classy Recurring Donor object is a Managed Package so I can’t create a Master detail field so I could create a Rollup Summary field. I need to create a field that will show the sequential number of each new record ie.1, 2, 3, 4, etc? I was told by a consultant that DLRS could make this possible. Is that true? Hoping the answer is yes.
August 24, 2017 at 1:08 am
It’s not really something it does really, this is not a rollup scenario. Try maybe looking at Process Builder and Flow?
Pingback: Swagger / Open API + Salesforce = LIKE | Andy in the Cloud
October 20, 2017 at 9:47 am
Andy – I wrote to you on Twitter when I first followed your steps above. All worked well. With a Swagger tutorial today, I build an API, and downloaded the JSON schema. I pasted that file (since I’m not hosting my app anywhere – just a publicly published Swagger API. SF does show the “Actions” page & saved the integration “complete.” But I don’t get the Apex anywhere; not available in Flow. If I look in detail at the Actions page, some I/O Parameters aren’t shown. Is there a tool to validate the JSON that Swagger site provided & I downloaded?
October 20, 2017 at 4:52 pm
Yes try https://swagger.io, if it is valid search for Belinda Wong from Salesforce on twitter and let her know
November 18, 2017 at 9:39 am
Andy: I posted before above – thanks for your reply. I am trying a new-to-me api (https://geocoder.opencagedata.com/api) for reverse geo-coding. They do have a swagger schema, which I have a Q’s about: 1. They host it at the ROOT of url. How do I indicate that as relative URL in the wizard? Simply “/” does not work. I did convert to JSON, and paste it, which reveals input/output parameters and the methods in the Integradtion [View Actions] – but I see no Apex entry for these in a brand new Flow I’ve made (similar to your ascii flow above). Any hints about why the integration would show actions, but no Apex would be visible in flow?
November 19, 2017 at 8:14 am
There is some issue with root, try removing the path entry in the schema before uploading.
November 19, 2017 at 8:25 am
Andy – thanks. I wanted to point to their (untouched) schema file – that they are hosting. I can edit their file and upload (minus path entry), but I certainly can’t edit theirs in place and refer to that. I’ll see if removing the schema reference from UPLOADED file helps any with the action visibility issue I reported.
November 19, 2017 at 8:47 am
Yes, that makes sense. Keep in mind this is in Beta. I have copied in the PM at Salesforce for this area, Belinda. You may want to share with her more details for her teams testing.
November 19, 2017 at 4:05 pm
Thank you very much for your help; I saw you cc the PM on Twitter. I really appreciate your help! I’d love to get this off the blocks and see how far I can push it! Thanks again.
November 21, 2017 at 4:04 pm
Yes it’s got great promise, it’s in Beta so let’s help Salesforce make it the best for GA. 👍🏻
Pingback: Swagger / Open API + Salesforce = LIKE – Kay Scott's Blog
Pingback: Around the Web – 20170728 – WIPDeveloper.com
Pingback: Extend Flows with Heroku Compute: An Event-Driven Pattern